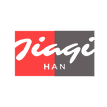
JIAQI HAN
La République
des Engrenages
La République des Engrenages is a strategy + tower defense game. Unlike previous tower defense games, players have nothing else to do but watch after setting up the tower. This game allows players to control their character to walk into the scene, balance their resources, and trigger mechanisms to shoot enemies.
-
3D Steampunk Game
-
Tower Defense
-
Strategy Game
-
ARPG Game
-
Work as Game Designer
-
Designed Art Style and Assets
-
Made with Unity, Blender, Mixamo, Photoshop
Digital Game Design
Individual Work
Summer 2023
Demo Video:
https://youtu.be/0wKQovGBZyA
Game Link:
Game Overview:
Welcome to the Republic of Gears—— a country ruled by a time machine towards new Electrical technology development.
Now, you are a Tesla soldier composed of gears and coils, trying to thwart the destructive invasion of adversaries from the old era, powered by steam. Your mission is to ensure the smooth operation of the wheel of time, preventing disruptions.
Activate some gear turrets with your electrical power to deliver more powerful blows to the enemy!
Use WASD to move, and press the left mouse button to set turrets and shoot. You can also see the guidance at the beginning of the game.
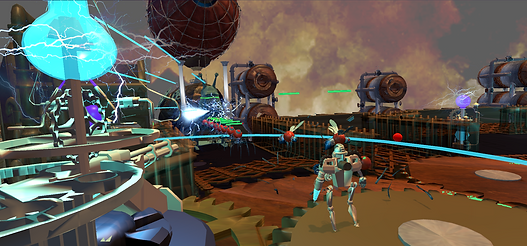
(Figure.Game screenshot with game concept expression)
Game Concept:
As Steampunk artist Kzuhiko Nakamura said, the essence of steampunk is the whims brought about by human regret and excessive mechanization.
The Industrial Revolution brought many urban problems, such as noise and environmental pollution. The emergence of clean energy, such as electricity, has brought a turnaround to everything.
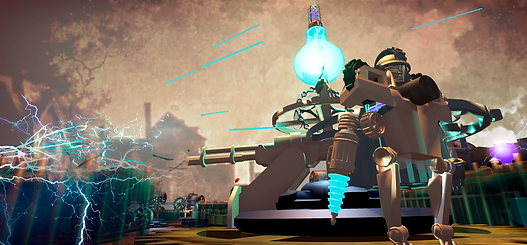
(Figure.Game screenshot with game concept expression)
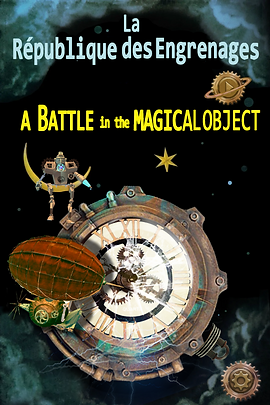
(Figure.The game poster, inspired by Le voyage dans la lune)
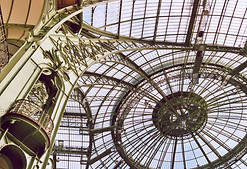
(Figure.Grand Palais in Paris)
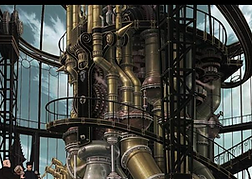
(Figure.Steam facility in Steamboy animation)
This game aims to express the conflict between the productivity powered by two different technologies (steam engine and electricity) in the historical context. In terms of environmental art, this game is influenced by many stylized steampunk art styles, such as Udagawa Yasuhito's insect biology punk, and architectural art, such as the Grand Palais in Paris and Crystal Palace in London.
The background music is from Steamboy animation. Their nostalgic yet powerful tone shares the same theme as the game.
Art and Forces:
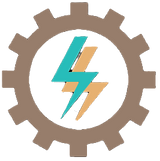
Player Culture Symbol
The protagonist symbolizes a more advanced productive force——electricity. Tesla soldier with electrified power represents an avatar of Teslapunk.

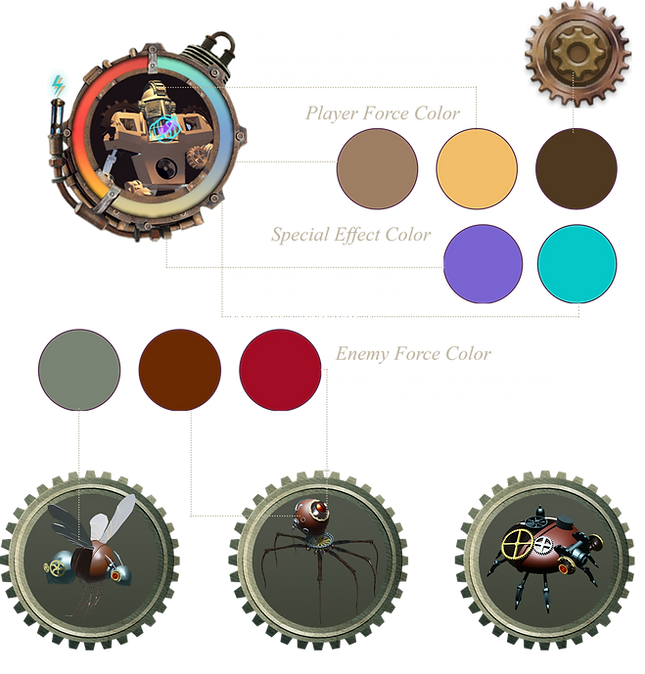
Enemy Culture Symbol
The enemies symbolize the old productive force powered by steam.
They look like mechanical insects, with glowing red, hot big eyes, leather bodies, and steam engines.
Wasp
Active and fond of attacking, symbolizing the noise problem caused by the First Industrial Revolution.
Speed: 1.6m/s
Blood Value: 800
Spider
Like to network everywhere and make the wheel of time get into trouble, symbolizing the backwardness of steam power productivity.
Speed: 0.8m/s
Blood Value: 1500
Scavenger Beetle/Bug
Some members may damage items, symbolizing the hygiene issue caused by the First Industrial Revolution.
Speed: 0.725m/s
Blood Value: 3000
Game Mechanics:
The realm of a time machine is powered by electricity, and three kinds of gear turrets can be activated to fire toward enemies.
Tower Defense
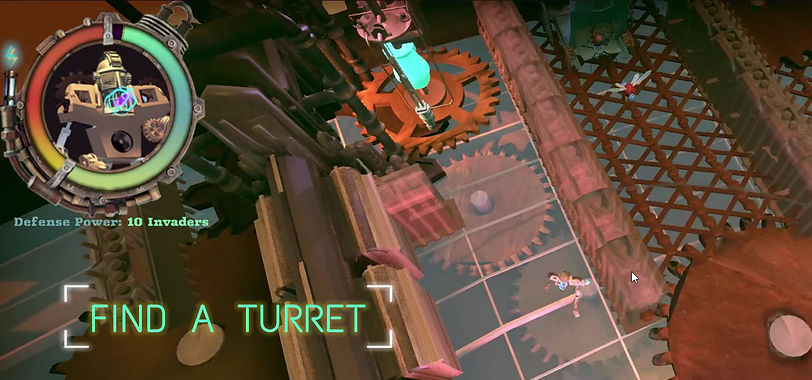
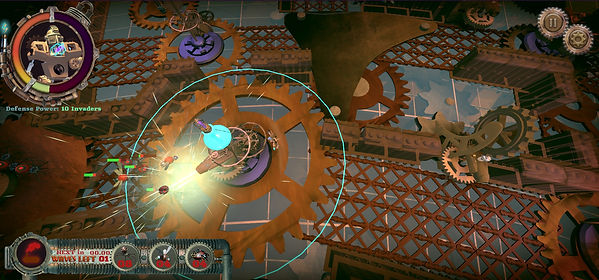
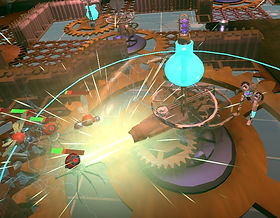
Heavy Gun
Heavy Gun supports range attacks. A heavy turret will exert an area to attack multiple enemies on the ground.
Heavy Gun
Energy cost to activate: 300
Attack Range: 8m
Fire Interval: 1.6s
Damage: 30
Damage Range: 10m


Saw
Saw reduces the enemy's health value when sweeping around.
Saw
Energy cost to activate: 150
Attack Range: 5m
Fire Interval: 1s
Damage: 20
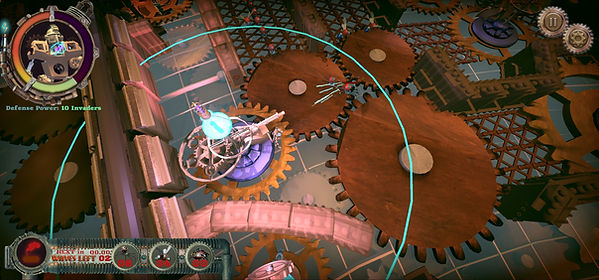
Laser Gun
With fast firing speed and long attack range, Laser Gun can only attack a single enemy.
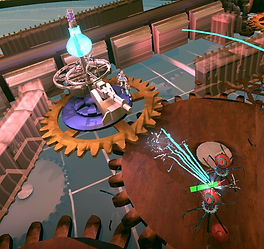
Laser Gun
Energy cost to activate: 250
Attack Range: 12m
Fire Interval: 0.7s
Damage: 50
Strategic Tower Defense
In order to retain players' interest in the later phase, I designed a controllable character——a Tesla soldier.
For most tower defense games, players have nothing else to do but watch after setting up the towers. This game allows players to control their character to walk into the scene, balance their resources, and trigger mechanisms to shoot enemies.
By controlling the Tesla soldier, the player can use WASD to move and press the left mouse button to set turrets and shoot.

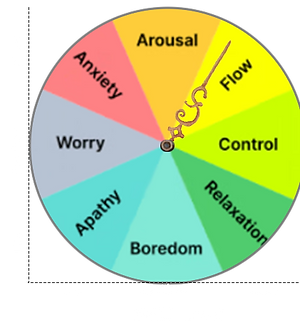
Tesla Soldier
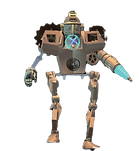
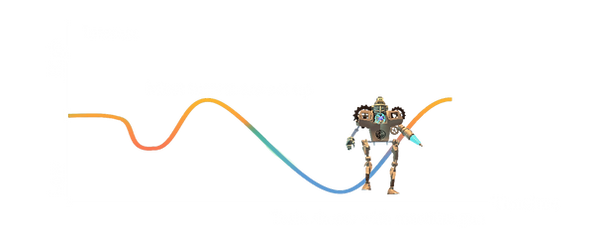
When most turrets are activated, Tesla can operate four fixed machine guns positioned near the endpoint of the level, making the game's results more controllable.

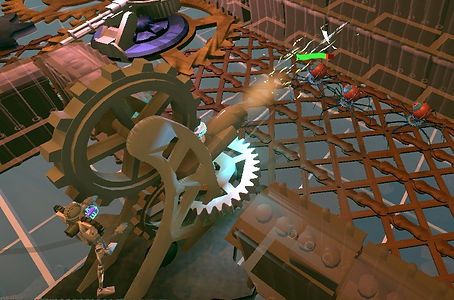
When Tesla approaches the machine gun, the control UI will pop up.
Tesla can strike enemies at the set frequency when he stands in a specific position behind the machine gun.
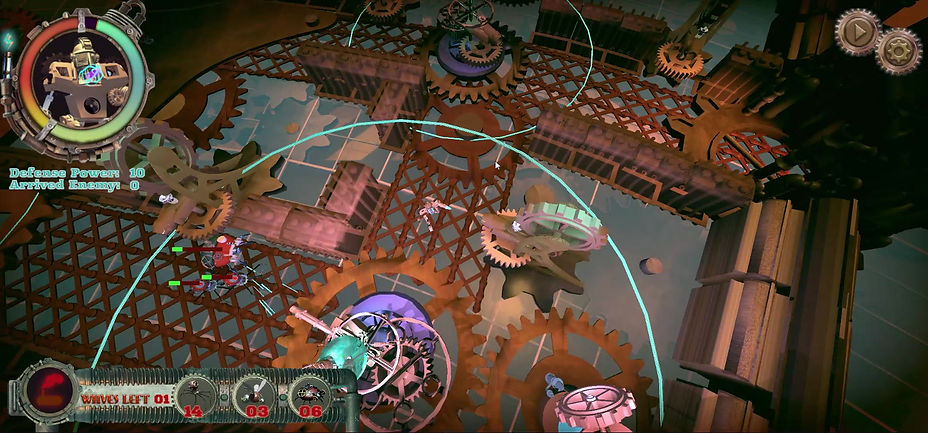

Charger helps Tesla recharge electricity.
Energy is an important resource!
When most turrets are activated, players can operate fixed machine guns through Tesla, with the machine guns positioned near the finish line, making the game's results more controllable.

Tesla consumes his electricity to active the gear turrets.
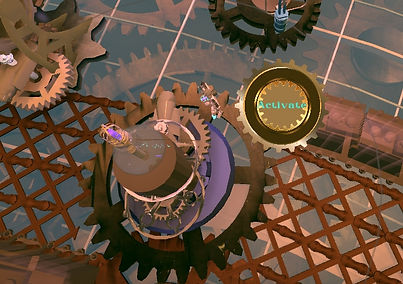
After a short period of activating time, the gear will be activated with the weapon available.
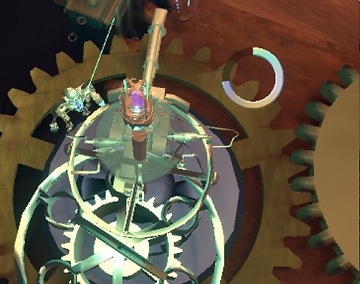
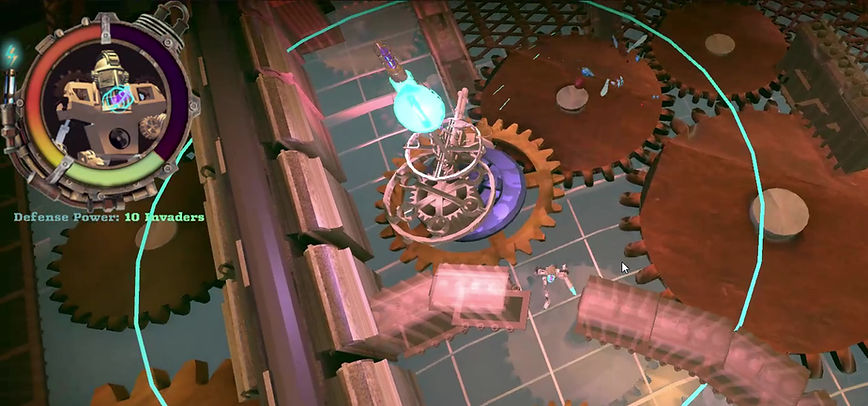
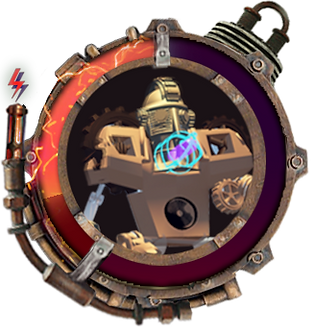
Since each activation of the defense turret and each shooting by a Tesla gun will consume the Tesla soldier's energy, there will be a punishment if the energy consumption is inefficient.
What if 0 Energy Value?
When the energy is too low, the UI icon will issue a red warning, reminding the Tesla solider that he needs to be charged; otherwise, he will be unable to unlock any turret. When the energy is zero, Tesla will be reassembled and resurrected after 3 seconds, which is a somewhat lengthy process, so players should try to avoid this situation as much as possible.
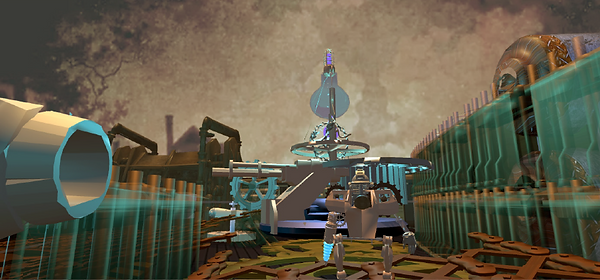
If he fails to find the charger for charging promptly or excessively consumes electricity, when the energy decreases to zero, Tesla will fall apart.
Then, he will be reassembled and resurrected after 3 seconds. Because each wave has a countdown, and the time spent on dismantling and restructuring is a waste, this time penalty mechanism is thus established.

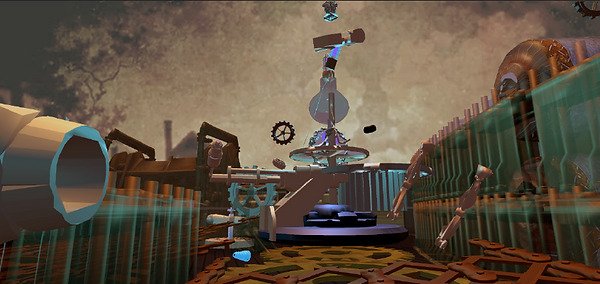

Level Design:
A typical level is shown in the figure, consisting of 4 different enemy routes, but the positions of the endpoint and starting point are the same. There will be a countdown for each wave in the game. The gate at the finish line can resist attacks from 10 enemies. When the 10th enemy reaches the finish line, the player fails.
A typical level is shown in the figure, consisting of 4 different enemy routes, but the positions of the endpoint and starting point are the same. There will be a countdown for each wave in the game. The gate at the finish line can resist attacks from 10 enemies. When the 10th enemy reaches the finish line, the player fails.

(Figure.Screenshot during playing game)
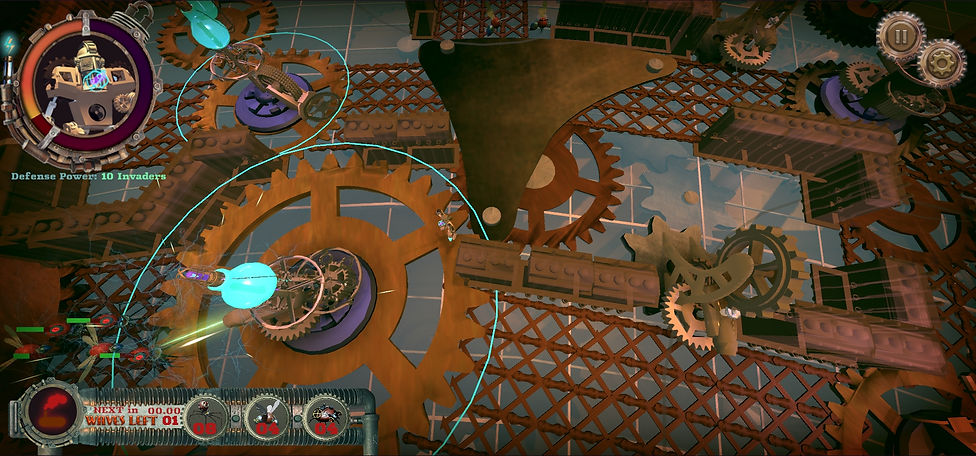
Technical:
Completed with the support from programmer Song Huang
Environmental Art
I referred to architectural art when designing the environmental elements, such as the Grand Palais in Paris and the Crystal Palace in London. The overall color tone of the game has been re-adjusted with post-process volume and post-process layer components.
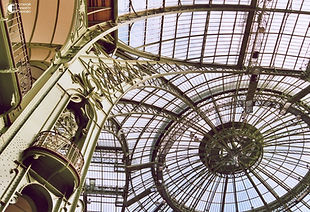
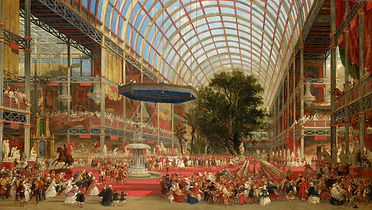
(Figure.Grand Palais in Paris)
(Figure.Crystal Palace in London)
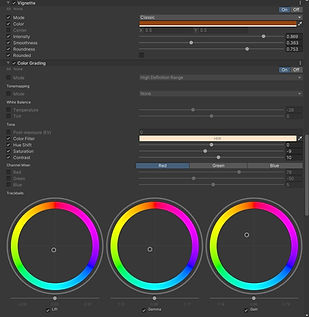
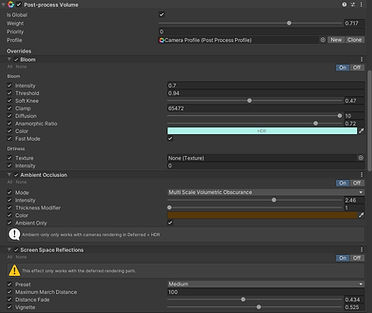
2023.10.03 Version:
Plan Design: The interactive gears are not easily distinguishable from the decorations.
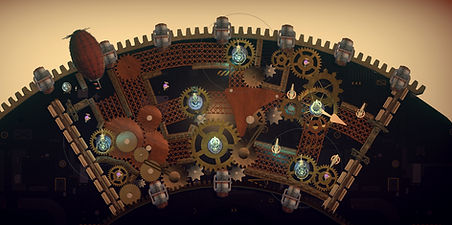
Vertical Design: All items are integrated into the same layer, so the player will be confused.
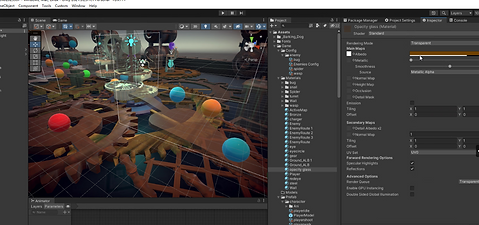
2023.10.30 Version:
Plan Design: I added one piece of delicate glass to cover some decorations. The gears are more golden in color.
Vertical Design: The interactable items and decorations are separated into different layers.
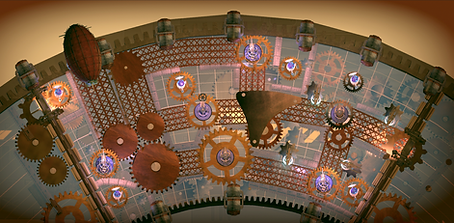
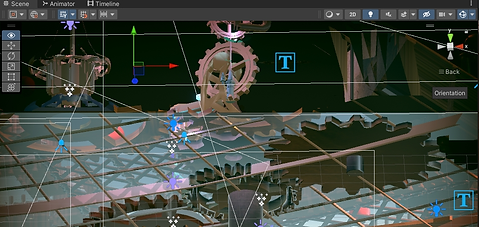
Interactable spatial UI
There are many interactive structures in the game, such as chargers, machine guns and turrets. In order to increase the usability of scripts, it is better to define a MonoBehaviour called StructureBehaviour, to control all the interactable structures which has a interactive button.
In order to make the scene clearer, the UI button only prompts when the player approaches them. So many structure shhould have colliders to test if the player is nearby or not. This UI is a spatial UI, it is 3D in the world but set up on Canvas and always face the main camera.
Each interactable button has three layers:
Progress Bar, Interact Button and Text.



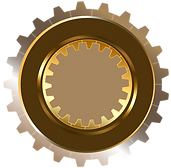
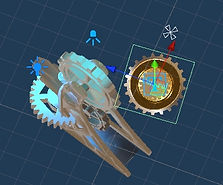

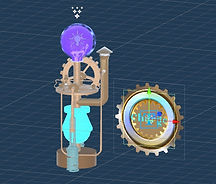
Progress Bar
Text
Interact Button
The flow chart of interactable UI is shown below:

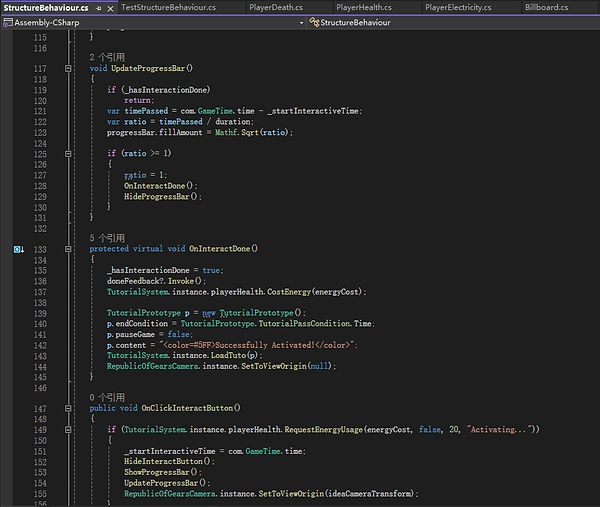
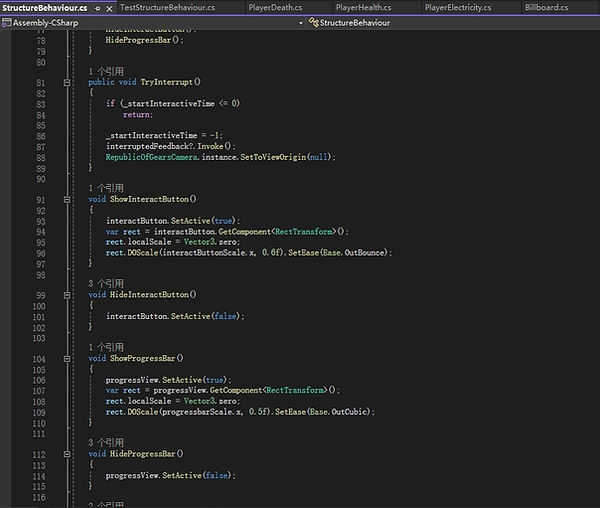
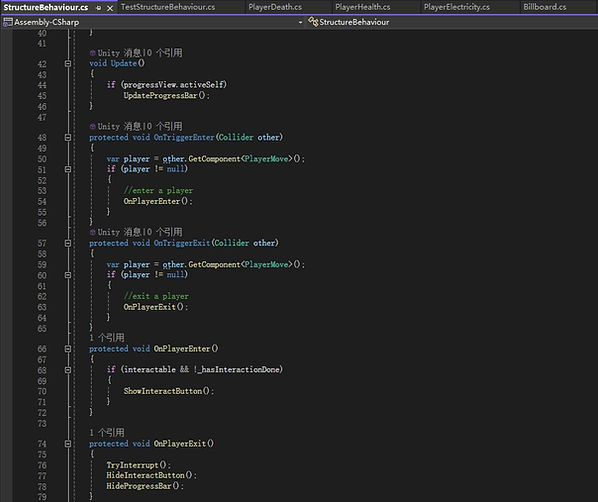
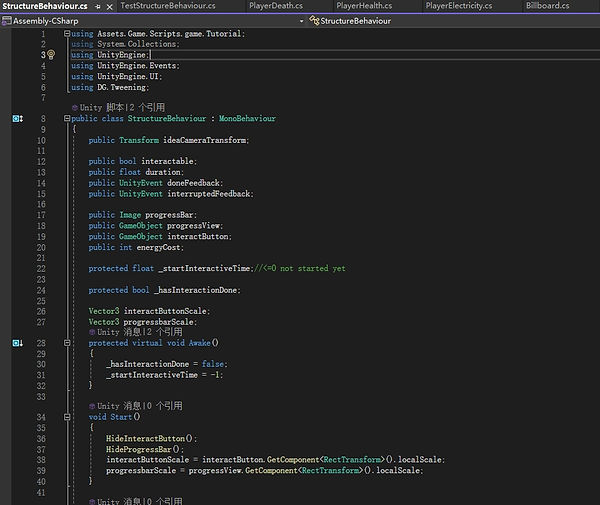
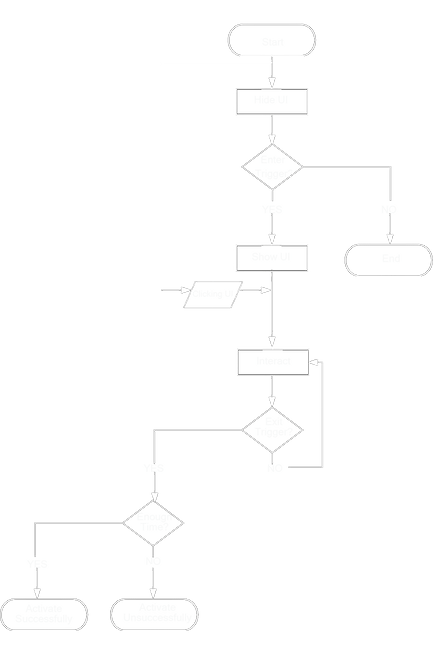
Program Structure
Program Framework
1.Player
Player controller (health)
Player movement (death)
Shooting controller
2.Level
Level setting (time, score indicator, etc)
Level UI controller (non-digetic UI, spatial UI)
Sound effect manager
Material Manager
Tutorial
3.Enemies
Enermy controller
Enermy routes
Enermy movement
Enermy config (blood, speed, prefab model)
4.Waves
Timer
Route information
5.Turret
Attack range drawer
Search target behaviour
Weapon
Rotate gun behaviour
VFX
Structure activation
6.Machine Gun
Interactive behavior of machine gun

The Implementation of the Enemy's Advance Route
Here is an example of a route, which consists of multiple route points. Each point helps the enemy to track key directions.

For a tower defense game, complexity should be considered in the programming since the enemies become more and more as time passes.
In order to make the enemy army not so costly to the program, this project applied rigidbody instead of a character controller to move and generated all enemies with prefab and configuration to complete automation of repetitive tasks.
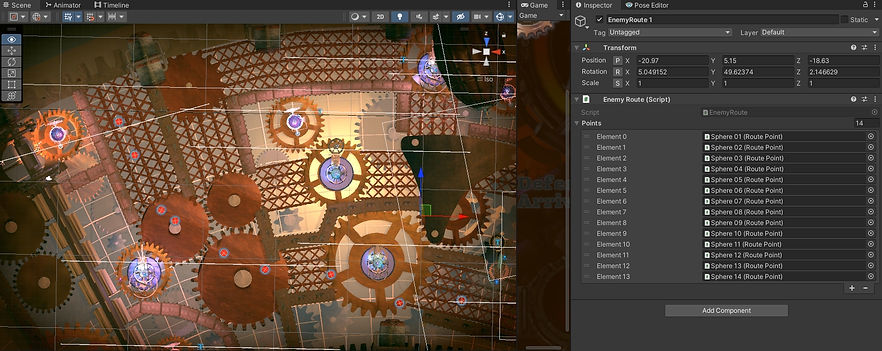

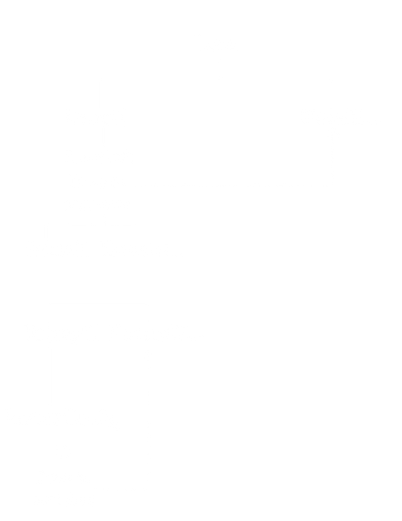
Coordination of Collision Detection between Gameobjects
1. "Has Reach" the route point:
The enemy's collider volume is relatively large and if it has not passed through a particular point because it is pushed away by other enemies, it becomes difficult to determine its path. In such cases, it is necessary to increase the tolerance value. Even if the enemy has not fully passed through the path point, it is still considered as having reached the point.



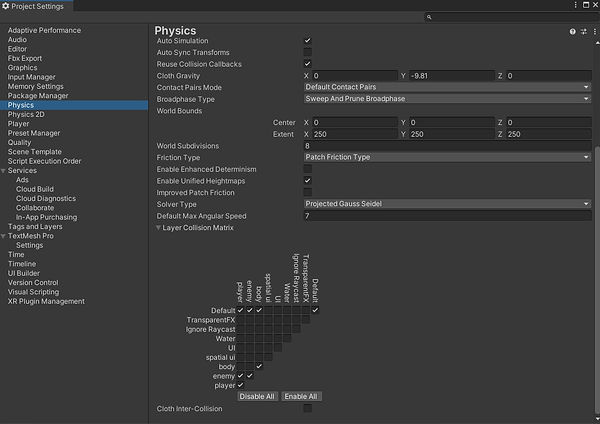
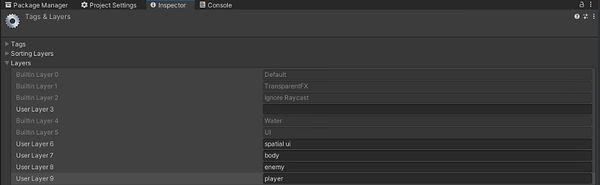

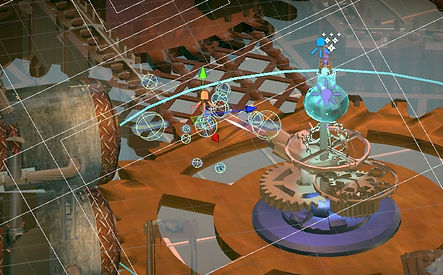
(Figure.The condition colliders in Unity)
2. Layer Collision Matrix:
When the enemy is blown into pieces, some parts of its body will fly to a larger range of space. The colliders on its body parts will push other enemies and the Tesla Soldier to deflect. So, in the project settings, more collision layers should be considered to ensure they will not exert unnecessary influence on each other.
Assets Production Workflow
1.Search for reference materials and generate ideal character design with Photoshop and Midjourney.
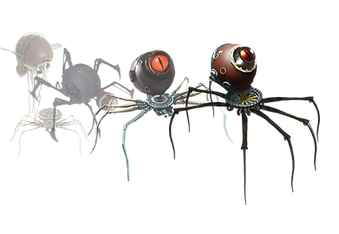

2. Build and assemble all models in Blender.
Enemies
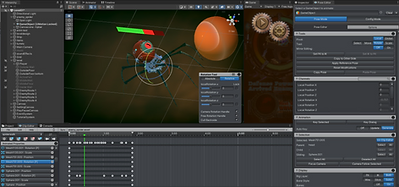
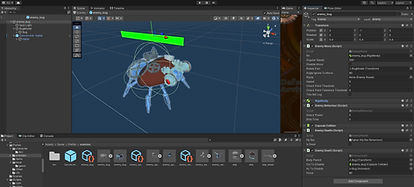
3. Generate bones for enemies through UMotion plugin in Unity.
4. To create enemies one by one, it is best to use prefabs for each kind of enemy.
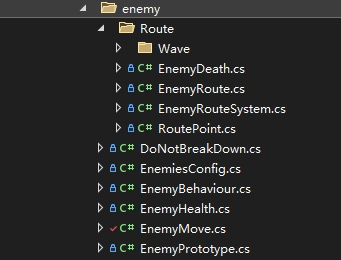
5. Link features like health points, speed with script to record the enemy's state.
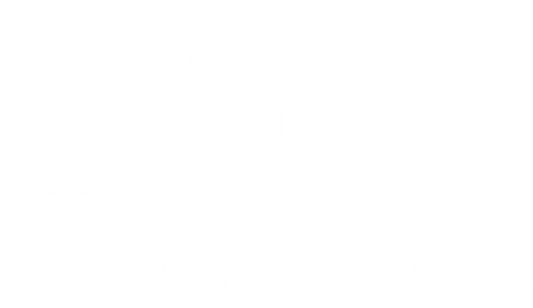
The configuration of each enemy prefab is shown below:
Tesla Soldier
Property:
The configuration of Tesla is shown on the right:
Motion:
In order to differentiate the robot's movements from those of humans, some code was added to change the angle of the protagonist's turn. This causes the Tesla soldier to turn first before starting to walk. If the player wants to move in a direction more than 45 degrees away from the protagonist's facing direction, he must press the directional key in order to continue moving forward.
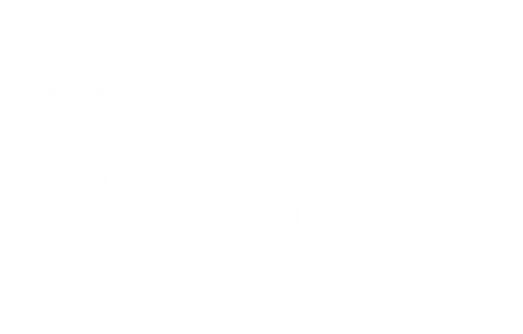
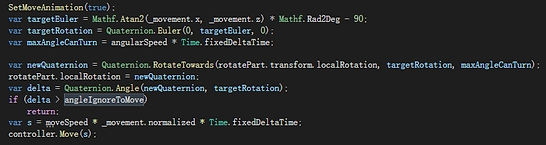
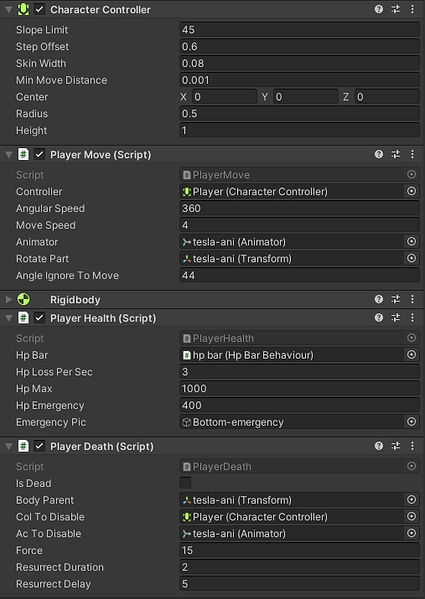

1.Search for reference materials and generate ideal character design with Photoshop and Midjourney.
2. Build and assemble all models in Blender.


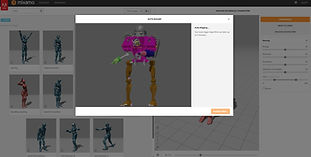.png)


3. Upload player model to Mixamo and download the animations.
4. Retarget the player's bones and generate bones for enemies through UMotion plugin in Unity.
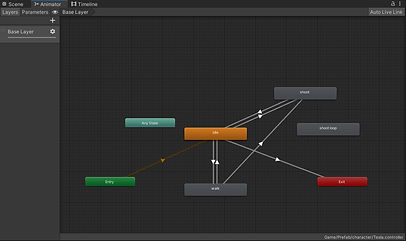
5. Script for animator and control the movement in Visual Studio with C#.
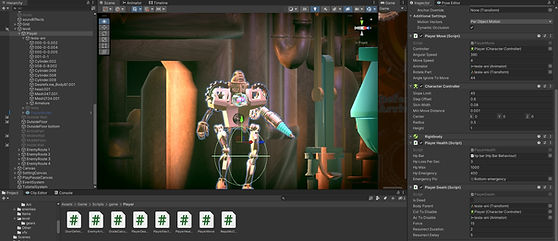
6. Link features like energy value, attack power with script to record the player's state.
Weapon Systems
Machine Gun
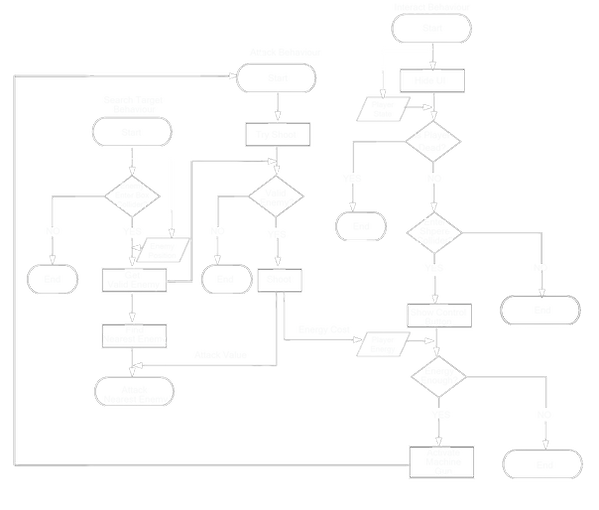
Shoot with Energy Cost:
In this game, the Tesla Soldier's energy functions as his "health points," the resource to trigger turrets, and the bullet to activate machine guns. Therefore, all actions in the game should be connected to the energy system to manage and track the player's energy value.
The program structure of the machine gun is shown on the right:
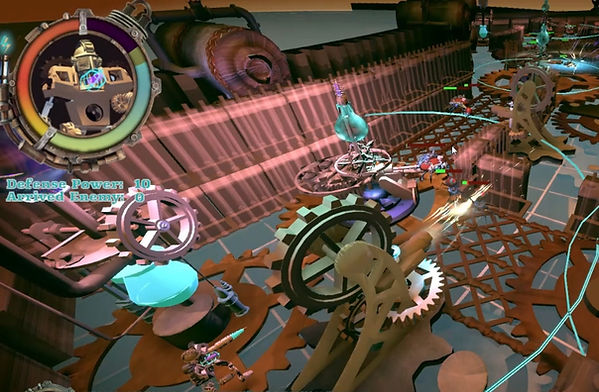
The energy circle is divided into two halves: the right half represents the energy level of 100%-40%, shown in green; while the left half represents the energy level of 40%-0%, shown in red. When the energy value falls below 40%, the UI will issue a red warning to remind the player to recharge the Tesla Soldier.
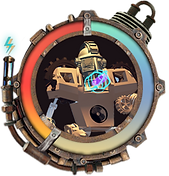
Full Energy

Energy Value <40%
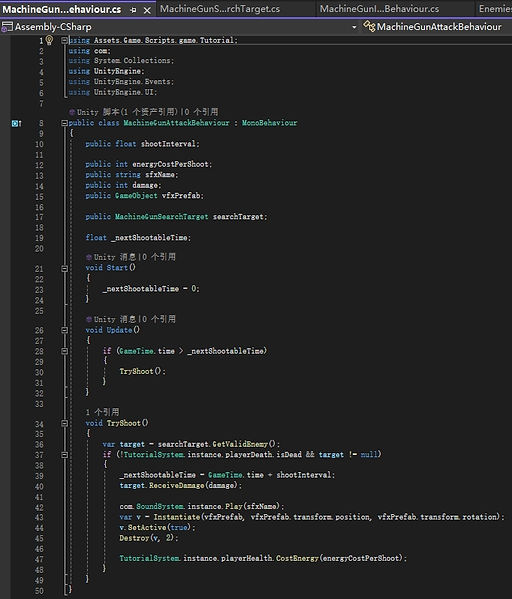
(Figure MachineGunAttackBehaviour.cs)
(Figure MachineGunAttackBehaviour.cs)
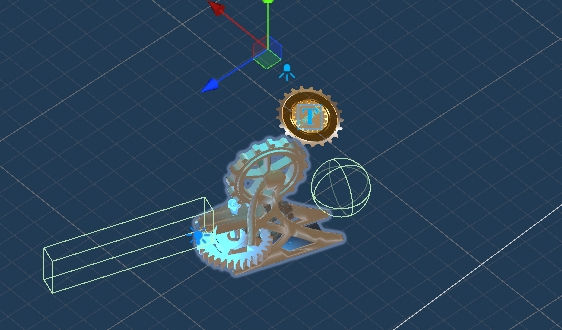
Collider Boxes:
To take control of the machine gun, two collider boxes are set.
Sphere Collider: To check valid firing position
The sphere collider tests if the player enters a certain position close enough to the machine gun to start shooting.
Box Collider: To check valid enemy
The box collider checks if there are any enemies within the firing range. If there are multiple enemies in the collision box, the machine gun will shoot the enemy with the smallest absolute distance.
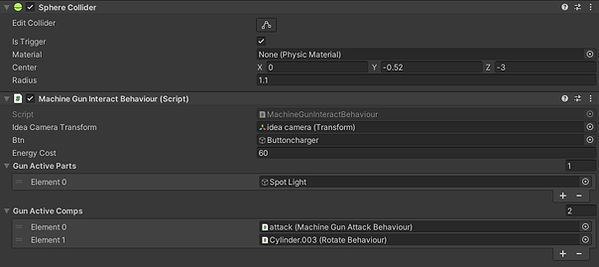
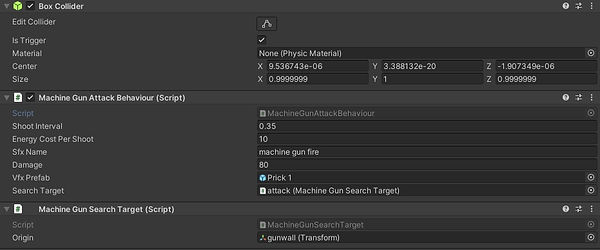
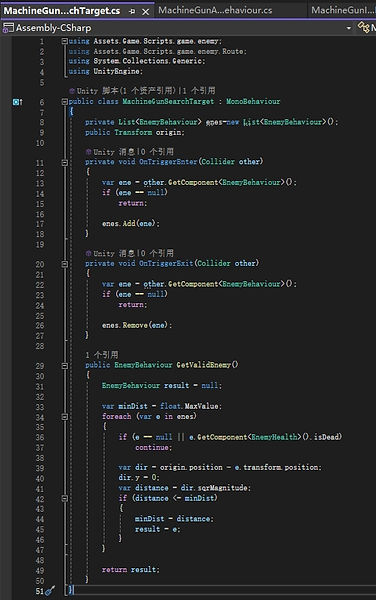
(Figure MachineGunSearchTarget.cs)
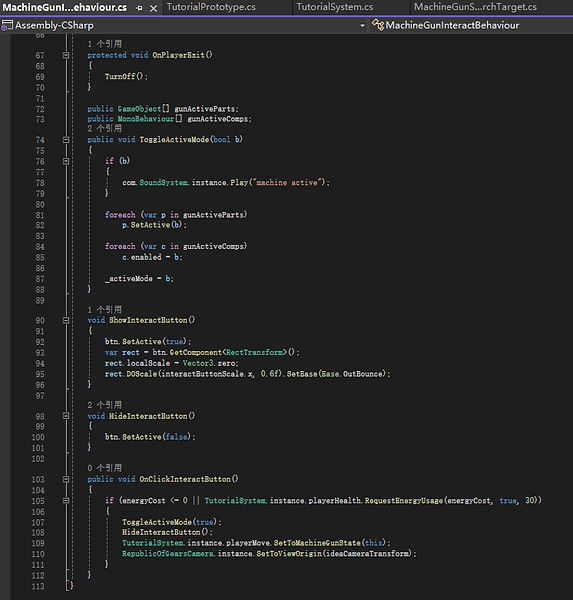
(Figure MachineGunInteractBehaviour.cs)
Turrets
In order to help players identify which turret is activated from a top-down perspective, I designed a small light that changes material. When the turret is activated, this light illuminates.
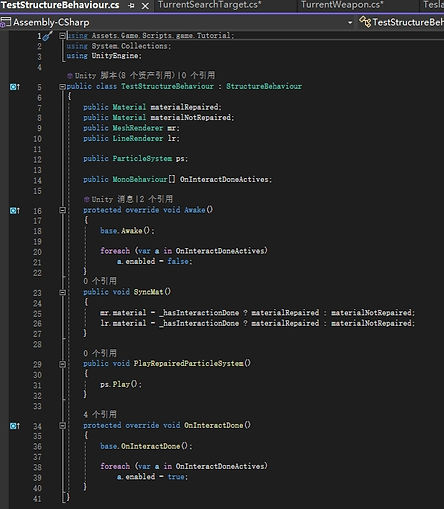

Turret Programmed System
The game contains three different types of turrets, but their functioning logic is identical. Every turret needs to perform three tasks: searching for the target, rotating the muzzle, and firing the weapon. To accomplish these tasks, three scripts are utilized, with each script responsible for one of the three tasks.

Before activation
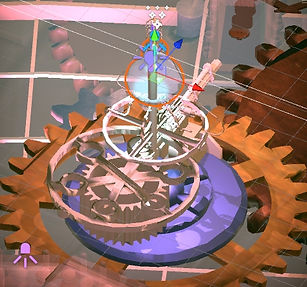

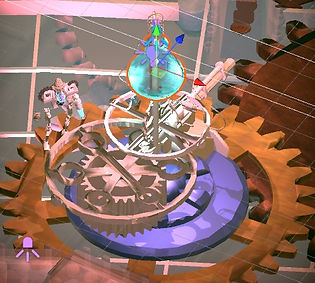
After activation
Target Searcher:
As enemies often march in groups, there are frequently multiple enemies within firing range. This program is designed to target the earliest-spawned enemy, making it suitable for attacking individual enemies.
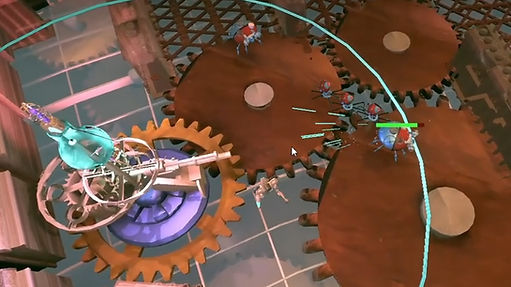

Muzzle Rotation:
The direction in which the muzzle rotates is determined by the position of the targeted enemy in the search results of the Target Searcher. To ensure a smooth rotation, an interpolation calculation is performed, which prevents the muzzle from suddenly jumping to the final position.

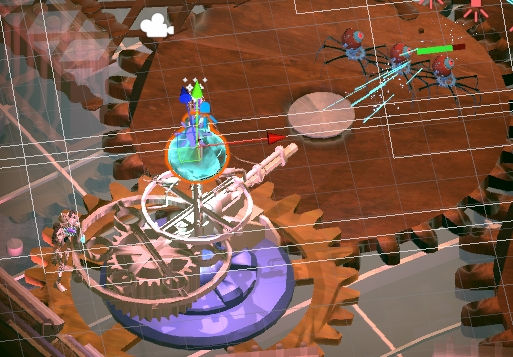
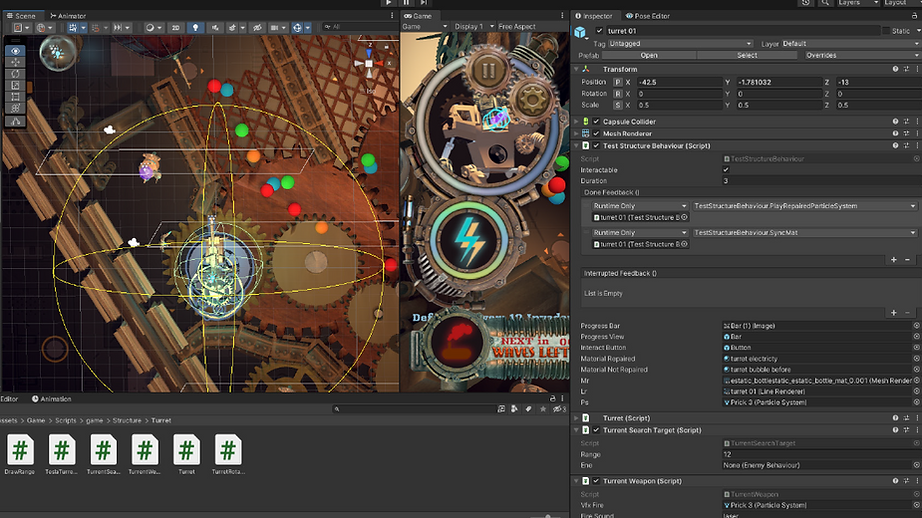
Turret Weapon:
Every time the turret fires, the program calls vfx and sound. For individual attacks, the turret fires at the earliest spawn enemy.

For group attacks, all enemies within a certain radius of the center of damage receive the damage (damageEffectiveRange). The damage value decreases from near to far, calculated using the Mathf function and the damage curve (Mathf. CailToInt (damage * damageCurve. Evaluate (distance/damageEffectiveRange).
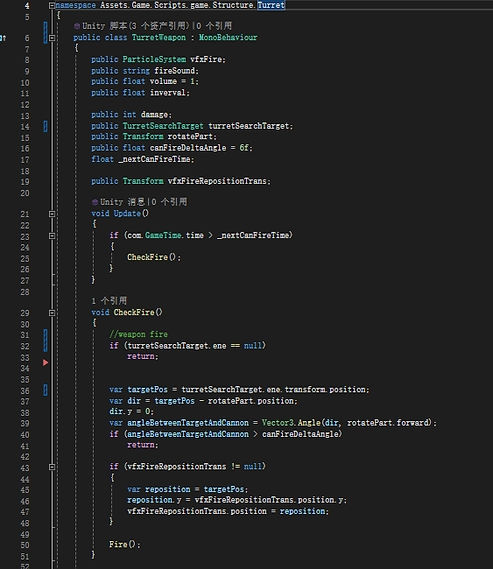
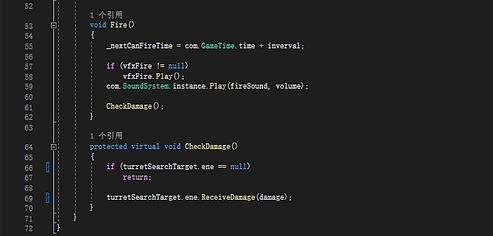
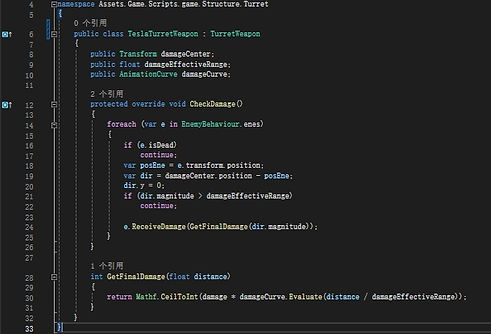
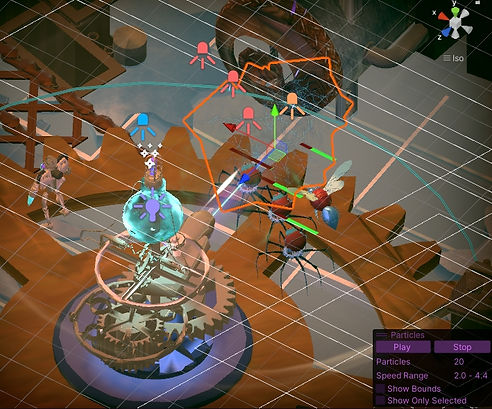